VR extension of PointerInputModule which supports gaze and controller pointing. More...
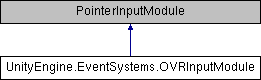
Public Types | |
enum | InputMode { InputMode.Mouse, InputMode.Buttons } |
Public Member Functions | |
override void | UpdateModule () |
override bool | IsModuleSupported () |
override bool | ShouldActivateModule () |
override void | ActivateModule () |
override void | DeactivateModule () |
override void | Process () |
Process this InputModule. Same as the StandaloneInputModule version, except that it calls ProcessMouseEvent twice, once for gaze pointers, and once for mouse pointers. More... | |
Public Attributes | |
Transform | rayTransform |
OVRCursor | m_Cursor |
OVRInput.Button | joyPadClickButton = OVRInput.Button.One |
KeyCode | gazeClickKey = KeyCode.Space |
bool | performSphereCastForGazepointer |
bool | useRightStickScroll = true |
float | rightStickDeadZone = 0.15f |
bool | useSwipeScroll = true |
float | swipeDragThreshold = 2 |
float | swipeDragScale = 1f |
bool | InvertSwipeXAxis = false |
OVRRaycaster | activeGraphicRaycaster |
float | angleDragThreshold = 1 |
Protected Member Functions | |
OVRInputModule () | |
void | CopyFromTo (OVRPointerEventData @from, OVRPointerEventData @to) |
Convenience function for cloning PointerEventData More... | |
new void | CopyFromTo (PointerEventData @from, PointerEventData @to) |
Convenience function for cloning PointerEventData More... | |
bool | GetPointerData (int id, out OVRPointerEventData data, bool create) |
new void | ClearSelection () |
Clear pointer state for both types of pointer More... | |
virtual MouseState | GetGazePointerData () |
State for a pointer controlled by a world space ray. E.g. gaze pointer More... | |
MouseState | GetCanvasPointerData () |
Get state for pointer which is a pointer moving in world space across the surface of a world space canvas. More... | |
Vector2 | SwipeAdjustedPosition (Vector2 originalPosition, PointerEventData pointerEvent) |
override void | ProcessDrag (PointerEventData pointerEvent) |
Exactly the same as the code from PointerInputModule, except that we call our own IsPointerMoving. More... | |
virtual PointerEventData.FramePressState | GetGazeButtonState () |
Get state of button corresponding to gaze pointer More... | |
Vector2 | GetExtraScrollDelta () |
Get extra scroll delta from gamepad More... | |
Protected Attributes | |
Dictionary< int, OVRPointerEventData > | m_VRRayPointerData = new Dictionary<int, OVRPointerEventData>() |
Properties | |
InputMode | inputMode [get] |
bool | allowActivationOnMobileDevice [get, set] |
float | inputActionsPerSecond [get, set] |
string | horizontalAxis [get, set] |
Name of the horizontal axis for movement (if axis events are used). More... | |
string | verticalAxis [get, set] |
Name of the vertical axis for movement (if axis events are used). More... | |
string | submitButton [get, set] |
string | cancelButton [get, set] |
Detailed Description
VR extension of PointerInputModule which supports gaze and controller pointing.
Member Enumeration Documentation
◆ InputMode
Constructor & Destructor Documentation
◆ OVRInputModule()
|
protected |
Member Function Documentation
◆ ActivateModule()
override void UnityEngine.EventSystems.OVRInputModule.ActivateModule | ( | ) |
◆ ClearSelection()
|
protected |
Clear pointer state for both types of pointer
◆ CopyFromTo() [1/2]
|
protected |
Convenience function for cloning PointerEventData
- Parameters
-
from Copy this value to to this object
◆ CopyFromTo() [2/2]
|
protected |
Convenience function for cloning PointerEventData
- Parameters
-
from Copy this value to to this object
◆ DeactivateModule()
override void UnityEngine.EventSystems.OVRInputModule.DeactivateModule | ( | ) |
◆ GetCanvasPointerData()
|
protected |
Get state for pointer which is a pointer moving in world space across the surface of a world space canvas.
- Returns
◆ GetExtraScrollDelta()
|
protected |
Get extra scroll delta from gamepad
◆ GetGazeButtonState()
|
protectedvirtual |
Get state of button corresponding to gaze pointer
- Returns
◆ GetGazePointerData()
|
protectedvirtual |
State for a pointer controlled by a world space ray. E.g. gaze pointer
- Returns
◆ GetPointerData()
|
protected |
◆ IsModuleSupported()
override bool UnityEngine.EventSystems.OVRInputModule.IsModuleSupported | ( | ) |
◆ Process()
override void UnityEngine.EventSystems.OVRInputModule.Process | ( | ) |
Process this InputModule. Same as the StandaloneInputModule version, except that it calls ProcessMouseEvent twice, once for gaze pointers, and once for mouse pointers.
◆ ProcessDrag()
|
protected |
Exactly the same as the code from PointerInputModule, except that we call our own IsPointerMoving.
This would also not be necessary if PointerEventData.IsPointerMoving was virtual
- Parameters
-
pointerEvent
◆ ShouldActivateModule()
override bool UnityEngine.EventSystems.OVRInputModule.ShouldActivateModule | ( | ) |
◆ SwipeAdjustedPosition()
|
protected |
◆ UpdateModule()
override void UnityEngine.EventSystems.OVRInputModule.UpdateModule | ( | ) |
Member Data Documentation
◆ activeGraphicRaycaster
OVRRaycaster UnityEngine.EventSystems.OVRInputModule.activeGraphicRaycaster |
◆ angleDragThreshold
float UnityEngine.EventSystems.OVRInputModule.angleDragThreshold = 1 |
◆ gazeClickKey
KeyCode UnityEngine.EventSystems.OVRInputModule.gazeClickKey = KeyCode.Space |
◆ InvertSwipeXAxis
bool UnityEngine.EventSystems.OVRInputModule.InvertSwipeXAxis = false |
◆ joyPadClickButton
OVRInput.Button UnityEngine.EventSystems.OVRInputModule.joyPadClickButton = OVRInput.Button.One |
◆ m_Cursor
OVRCursor UnityEngine.EventSystems.OVRInputModule.m_Cursor |
◆ m_VRRayPointerData
|
protected |
◆ performSphereCastForGazepointer
bool UnityEngine.EventSystems.OVRInputModule.performSphereCastForGazepointer |
◆ rayTransform
Transform UnityEngine.EventSystems.OVRInputModule.rayTransform |
◆ rightStickDeadZone
float UnityEngine.EventSystems.OVRInputModule.rightStickDeadZone = 0.15f |
◆ swipeDragScale
float UnityEngine.EventSystems.OVRInputModule.swipeDragScale = 1f |
◆ swipeDragThreshold
float UnityEngine.EventSystems.OVRInputModule.swipeDragThreshold = 2 |
◆ useRightStickScroll
bool UnityEngine.EventSystems.OVRInputModule.useRightStickScroll = true |
◆ useSwipeScroll
bool UnityEngine.EventSystems.OVRInputModule.useSwipeScroll = true |
Property Documentation
◆ allowActivationOnMobileDevice
|
getset |
◆ cancelButton
|
getset |
◆ horizontalAxis
|
getset |
Name of the horizontal axis for movement (if axis events are used).
◆ inputActionsPerSecond
|
getset |
◆ inputMode
|
get |
◆ submitButton
|
getset |
◆ verticalAxis
|
getset |
Name of the vertical axis for movement (if axis events are used).
The documentation for this class was generated from the following file:
- Oculus/VR/Scripts/Util/OVRInputModule.cs