This simple Chat UI demonstrate basics usages of the Chat Api More...
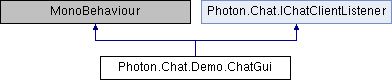
Public Member Functions | |
void | Start () |
void | Connect () |
void | OnDestroy () |
To avoid that the Editor becomes unresponsive, disconnect all Photon connections in OnDestroy. More... | |
void | OnApplicationQuit () |
To avoid that the Editor becomes unresponsive, disconnect all Photon connections in OnApplicationQuit. More... | |
void | Update () |
void | OnEnterSend () |
void | OnClickSend () |
void | PostHelpToCurrentChannel () |
void | DebugReturn (ExitGames.Client.Photon.DebugLevel level, string message) |
void | OnConnected () |
Client is connected now. More... | |
void | OnDisconnected () |
Disconnection happened. More... | |
void | OnChatStateChange (ChatState state) |
The ChatClient's state changed. Usually, OnConnected and OnDisconnected are the callbacks to react to. More... | |
void | OnSubscribed (string[] channels, bool[] results) |
Result of Subscribe operation. Returns subscription result for every requested channel name. More... | |
void | OnSubscribed (string channel, string[] users, Dictionary< object, object > properties) |
void | OnUnsubscribed (string[] channels) |
Result of Unsubscribe operation. Returns for channel name if the channel is now unsubscribed. More... | |
void | OnGetMessages (string channelName, string[] senders, object[] messages) |
Notifies app that client got new messages from server Number of senders is equal to number of messages in 'messages'. Sender with number '0' corresponds to message with number '0', sender with number '1' corresponds to message with number '1' and so on More... | |
void | OnPrivateMessage (string sender, object message, string channelName) |
Notifies client about private message More... | |
void | OnStatusUpdate (string user, int status, bool gotMessage, object message) |
New status of another user (you get updates for users set in your friends list). More... | |
void | OnUserSubscribed (string channel, string user) |
A user has subscribed to a public chat channel More... | |
void | OnUserUnsubscribed (string channel, string user) |
A user has unsubscribed from a public chat channel More... | |
void | OnChannelPropertiesChanged (string channel, string userId, Dictionary< object, object > properties) |
void | OnUserPropertiesChanged (string channel, string targetUserId, string senderUserId, Dictionary< object, object > properties) |
void | OnErrorInfo (string channel, string error, object data) |
void | AddMessageToSelectedChannel (string msg) |
void | ShowChannel (string channelName) |
void | OpenDashboard () |
![]() | |
void | DebugReturn (DebugLevel level, string message) |
All debug output of the library will be reported through this method. Print it or put it in a buffer to use it on-screen. More... | |
Public Attributes | |
string [] | ChannelsToJoinOnConnect |
string [] | FriendsList |
int | HistoryLengthToFetch |
ChatClient | chatClient |
GameObject | missingAppIdErrorPanel |
GameObject | ConnectingLabel |
RectTransform | ChatPanel |
GameObject | UserIdFormPanel |
InputField | InputFieldChat |
Text | CurrentChannelText |
Toggle | ChannelToggleToInstantiate |
GameObject | FriendListUiItemtoInstantiate |
bool | ShowState = true |
GameObject | Title |
Text | StateText |
Text | UserIdText |
int | TestLength = 2048 |
Properties | |
string | UserName [get, set] |
Detailed Description
This simple Chat UI demonstrate basics usages of the Chat Api
The ChatClient basically lets you create any number of channels.
some friends are already set in the Chat demo "DemoChat-Scene", 'Joe', 'Jane' and 'Bob', simply log with them so that you can see the status changes in the Interface
Workflow: Create ChatClient, Connect to a server with your AppID, Authenticate the user (apply a unique name,) and subscribe to some channels. Subscribe a channel before you publish to that channel!
Note: Don't forget to call ChatClient.Service() on Update to keep the Chatclient operational.
Member Function Documentation
◆ AddMessageToSelectedChannel()
void Photon.Chat.Demo.ChatGui.AddMessageToSelectedChannel | ( | string | msg | ) |
◆ Connect()
void Photon.Chat.Demo.ChatGui.Connect | ( | ) |
◆ DebugReturn()
void Photon.Chat.Demo.ChatGui.DebugReturn | ( | ExitGames.Client.Photon.DebugLevel | level, |
string | message | ||
) |
◆ OnApplicationQuit()
void Photon.Chat.Demo.ChatGui.OnApplicationQuit | ( | ) |
To avoid that the Editor becomes unresponsive, disconnect all Photon connections in OnApplicationQuit.
◆ OnChannelPropertiesChanged()
void Photon.Chat.Demo.ChatGui.OnChannelPropertiesChanged | ( | string | channel, |
string | userId, | ||
Dictionary< object, object > | properties | ||
) |
◆ OnChatStateChange()
void Photon.Chat.Demo.ChatGui.OnChatStateChange | ( | ChatState | state | ) |
The ChatClient's state changed. Usually, OnConnected and OnDisconnected are the callbacks to react to.
- Parameters
-
state The new state.
Implements Photon.Chat.IChatClientListener.
◆ OnClickSend()
void Photon.Chat.Demo.ChatGui.OnClickSend | ( | ) |
◆ OnConnected()
void Photon.Chat.Demo.ChatGui.OnConnected | ( | ) |
Client is connected now.
Clients have to be connected before they can send their state, subscribe to channels and send any messages.
Implements Photon.Chat.IChatClientListener.
◆ OnDestroy()
void Photon.Chat.Demo.ChatGui.OnDestroy | ( | ) |
To avoid that the Editor becomes unresponsive, disconnect all Photon connections in OnDestroy.
◆ OnDisconnected()
void Photon.Chat.Demo.ChatGui.OnDisconnected | ( | ) |
Disconnection happened.
Implements Photon.Chat.IChatClientListener.
◆ OnEnterSend()
void Photon.Chat.Demo.ChatGui.OnEnterSend | ( | ) |
◆ OnErrorInfo()
void Photon.Chat.Demo.ChatGui.OnErrorInfo | ( | string | channel, |
string | error, | ||
object | data | ||
) |
◆ OnGetMessages()
void Photon.Chat.Demo.ChatGui.OnGetMessages | ( | string | channelName, |
string [] | senders, | ||
object [] | messages | ||
) |
Notifies app that client got new messages from server Number of senders is equal to number of messages in 'messages'. Sender with number '0' corresponds to message with number '0', sender with number '1' corresponds to message with number '1' and so on
- Parameters
-
channelName channel from where messages came senders list of users who sent messages messages list of messages it self
Implements Photon.Chat.IChatClientListener.
◆ OnPrivateMessage()
void Photon.Chat.Demo.ChatGui.OnPrivateMessage | ( | string | sender, |
object | message, | ||
string | channelName | ||
) |
Notifies client about private message
- Parameters
-
sender user who sent this message message message it self channelName channelName for private messages (messages you sent yourself get added to a channel per target username)
Implements Photon.Chat.IChatClientListener.
◆ OnStatusUpdate()
void Photon.Chat.Demo.ChatGui.OnStatusUpdate | ( | string | user, |
int | status, | ||
bool | gotMessage, | ||
object | message | ||
) |
New status of another user (you get updates for users set in your friends list).
- Parameters
-
user Name of the user. status New status of that user. gotMessage True if the status contains a message you should cache locally. False: This status update does not include a message (keep any you have). message Message that user set.
Implements Photon.Chat.IChatClientListener.
◆ OnSubscribed() [1/2]
void Photon.Chat.Demo.ChatGui.OnSubscribed | ( | string [] | channels, |
bool [] | results | ||
) |
Result of Subscribe operation. Returns subscription result for every requested channel name.
If multiple channels sent in Subscribe operation, OnSubscribed may be called several times, each call with part of sent array or with single channel in "channels" parameter. Calls order and order of channels in "channels" parameter may differ from order of channels in "channels" parameter of Subscribe operation.
- Parameters
-
channels Array of channel names. results Per channel result if subscribed.
Implements Photon.Chat.IChatClientListener.
◆ OnSubscribed() [2/2]
void Photon.Chat.Demo.ChatGui.OnSubscribed | ( | string | channel, |
string [] | users, | ||
Dictionary< object, object > | properties | ||
) |
◆ OnUnsubscribed()
void Photon.Chat.Demo.ChatGui.OnUnsubscribed | ( | string [] | channels | ) |
Result of Unsubscribe operation. Returns for channel name if the channel is now unsubscribed.
If multiple channels sent in Unsubscribe operation, OnUnsubscribed may be called several times, each call with part of sent array or with single channel in "channels" parameter. Calls order and order of channels in "channels" parameter may differ from order of channels in "channels" parameter of Unsubscribe operation.
- Parameters
-
channels Array of channel names that are no longer subscribed.
Implements Photon.Chat.IChatClientListener.
◆ OnUserPropertiesChanged()
void Photon.Chat.Demo.ChatGui.OnUserPropertiesChanged | ( | string | channel, |
string | targetUserId, | ||
string | senderUserId, | ||
Dictionary< object, object > | properties | ||
) |
◆ OnUserSubscribed()
void Photon.Chat.Demo.ChatGui.OnUserSubscribed | ( | string | channel, |
string | user | ||
) |
A user has subscribed to a public chat channel
- Parameters
-
channel Name of the chat channel user UserId of the user who subscribed
Implements Photon.Chat.IChatClientListener.
◆ OnUserUnsubscribed()
void Photon.Chat.Demo.ChatGui.OnUserUnsubscribed | ( | string | channel, |
string | user | ||
) |
A user has unsubscribed from a public chat channel
- Parameters
-
channel Name of the chat channel user UserId of the user who unsubscribed
Implements Photon.Chat.IChatClientListener.
◆ OpenDashboard()
void Photon.Chat.Demo.ChatGui.OpenDashboard | ( | ) |
◆ PostHelpToCurrentChannel()
void Photon.Chat.Demo.ChatGui.PostHelpToCurrentChannel | ( | ) |
◆ ShowChannel()
void Photon.Chat.Demo.ChatGui.ShowChannel | ( | string | channelName | ) |
◆ Start()
void Photon.Chat.Demo.ChatGui.Start | ( | ) |
◆ Update()
void Photon.Chat.Demo.ChatGui.Update | ( | ) |
Member Data Documentation
◆ ChannelsToJoinOnConnect
string [] Photon.Chat.Demo.ChatGui.ChannelsToJoinOnConnect |
◆ ChannelToggleToInstantiate
Toggle Photon.Chat.Demo.ChatGui.ChannelToggleToInstantiate |
◆ chatClient
ChatClient Photon.Chat.Demo.ChatGui.chatClient |
◆ ChatPanel
RectTransform Photon.Chat.Demo.ChatGui.ChatPanel |
◆ ConnectingLabel
GameObject Photon.Chat.Demo.ChatGui.ConnectingLabel |
◆ CurrentChannelText
Text Photon.Chat.Demo.ChatGui.CurrentChannelText |
◆ FriendListUiItemtoInstantiate
GameObject Photon.Chat.Demo.ChatGui.FriendListUiItemtoInstantiate |
◆ FriendsList
string [] Photon.Chat.Demo.ChatGui.FriendsList |
◆ HistoryLengthToFetch
int Photon.Chat.Demo.ChatGui.HistoryLengthToFetch |
◆ InputFieldChat
InputField Photon.Chat.Demo.ChatGui.InputFieldChat |
◆ missingAppIdErrorPanel
GameObject Photon.Chat.Demo.ChatGui.missingAppIdErrorPanel |
◆ ShowState
bool Photon.Chat.Demo.ChatGui.ShowState = true |
◆ StateText
Text Photon.Chat.Demo.ChatGui.StateText |
◆ TestLength
int Photon.Chat.Demo.ChatGui.TestLength = 2048 |
◆ Title
GameObject Photon.Chat.Demo.ChatGui.Title |
◆ UserIdFormPanel
GameObject Photon.Chat.Demo.ChatGui.UserIdFormPanel |
◆ UserIdText
Text Photon.Chat.Demo.ChatGui.UserIdText |
Property Documentation
◆ UserName
|
getset |
The documentation for this class was generated from the following file:
- 3rd-Party/Photon/PhotonChat/Demos/DemoChat/ChatGui.cs